If it is true, Matcher returns only those matches with value (i,j) such that i-th descriptor in set A has j-th descriptor in set B as the best match and vice-versa. print(points[0]) above give the next output, as example: So first of all, let'S look at your print, it says that points[0] is, You can unpack it like you did with the circle and then do the tuple. We can draw lines, polylines etc using opencv methods on different images. I was wondering is this is possible with some built-in way. Yes, this will work but the points must stay in a variable. A piecewise-linear curve is used to approximate the elliptic arc boundary. Angle between the subsequent polyline vertices. We will see how to match features in one image with others. We can also draw closed polyline as polygon, we just change is closed to True. Otherwise, this indicates that a filled ellipse sector is to be drawn. Like we used cv.drawKeypoints() to draw keypoints, cv.drawMatches() helps us to draw the matches. What is the symbol (which looks similar to an equals sign) called? pts: Array of polygonal curves. Why do people write "#!/usr/bin/env python" on the first line of a Python script? Can you still use Commanders Strike if the only attack available to forego is an attack against an ally? We sort them in ascending order of their distances so that best matches (with low distance) come to front. Here, we will see a simple example on how to match features between two images. Starting angle of the elliptic arc in degrees. It defines the approximation accuracy. In this article, we will discuss how to draw a line using OpenCV in C++. The image rectangle is Rect(0, 0, imgSize.width, imgSize.height) . Array of polygons where each polygon is represented as an array of points. Syntax: line (img, pt1, pt2, color, thickness, lineType, shift) Parameters: img: This is the image file. In this post we worked on drawing lines on images using cv2.line () and cv2.arrowedLine () method. Draws a simple or thick elliptic arc or fills an ellipse sector. We will first add the coordinates of the point we clicked in the points list. Indeed, when art emphasizes edges and pose, it often seems to convey the idea of an archetype, such as Rodin's . The function line draws the line segment between pt1 and pt2 points in the image. Polylines Polylines create lines for a list of points. opencv c++ asked Dec 15 '13 mahsa 58 6 updated Dec 20 '13 Moster 1716 5 24 If I want to draw a line between (center.x , center.y)in the current frame and (center.x , center.y) in the previous frame..should I every time that the code runs keep it in a vector and then take them two by two and use cvPoint () and cvLine () ? That is, the two features in both sets should match each other. Thickness of lines the contours are drawn with. Is "I didn't think it was serious" usually a good defence against "duty to rescue"? Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. How do I create a directory, and any missing parent directories? To draw a straight line between two points on an image we can use the OpenCV cv2.line(). A video is, after all, just a series of images. We will add this function as callback to mouse events. Then we will create a small dot to show the point where we clicked the image. Symbols that cannot be rendered using the specified font are replaced by question marks. [W / 4, 3 * W / 8], [13 * W / 32, 3 * W / 8], [5 * W / 16, 13 * W / 16], [W / 4, 13 * W / 16]], np.int32), atom_image = np.zeros(size, dtype=np.uint8), rook_image = np.zeros(size, dtype=np.uint8), my_filled_circle(atom_image, (W // 2, W // 2)), my_line(rook_image, (0, 15 * W // 16), (W, 15 * W // 16)), my_line(rook_image, (W // 4, 7 * W // 8), (W // 4, W)), my_line(rook_image, (W // 2, 7 * W // 8), (W // 2, W)), my_line(rook_image, (3 * W // 4, 7 * W // 8), (3 * W // 4, W)), # cv.polylines(img, [ppt], True, (255, 0, 255), line_type). Thickness of the lines used to draw a text. ( The images are /samples/data/box.png and /samples/data/box_in_scene.png). acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Data Structures & Algorithms in JavaScript, Data Structure & Algorithm-Self Paced(C++/JAVA), Full Stack Development with React & Node JS(Live), Android App Development with Kotlin(Live), Python Backend Development with Django(Live), DevOps Engineering - Planning to Production, GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Interview Preparation For Software Developers. I'm looking for a way to get the end points of a thin contour extracted from a Canny edge detection. import numpy as np import cv2 as cv # Create a black image img = np.zeros ( (512,512,3), np.uint8) # Draw a diagonal blue line with thickness of 5 px it returns false if the line segment is completely outside the rectangle. If it is negative (for example, thickness=. In the following snippet, the cv2.setMouseCallback function captures the mouse events and then appends the position of the mouse click when the left mouse button is pressed and stores the coordinates of the new point in the points list. OpenCV-Python is a library of Python bindings designed to solve computer vision problems. need cols we took [1]. But if we have more than two points and we need to draw a lot of lines between two points, for this cv2 line method can be used but for performance we can use polylines method from opencv. Bottom-left corner of the text string in the image. Draw a filled polygon by using the OpenCV function fillPoly () OpenCV Theory For this tutorial, we will heavily use two structures: cv::Point and cv::Scalar : Point It represents a 2D point, specified by its image coordinates and . He also rips off an arm to use as a sword. tuple with the image details as (rows, cols, channels), since we But we need to have those coordinates with us. (Python + opencv 3 . acknowledge that you have read and understood our, Data Structure & Algorithm Classes (Live), Data Structures & Algorithms in JavaScript, Data Structure & Algorithm-Self Paced(C++/JAVA), Full Stack Development with React & Node JS(Live), Android App Development with Kotlin(Live), Python Backend Development with Django(Live), DevOps Engineering - Planning to Production, GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Perspective Transformation Python OpenCV, Top 50+ Python Interview Questions & Answers (Latest 2023), Face Detection using Python and OpenCV with webcam, Adding new column to existing DataFrame in Pandas, How to get column names in Pandas dataframe, Reading and Writing to text files in Python, Python program to convert a list to string. The function cv::drawMarker draws a marker on a given position in the image. By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. Draw line between two given points (OpenCV, Python), How a top-ranked engineering school reimagined CS curriculum (Ep. We make use of First and third party cookies to improve our user experience. I need to create a polygon with these curves and fill them with white like the output below : import cv2 from google.colab.patches import cv2_imshow from google.colab import drive import numpy as np filePath = '/gdrive/My Drive/teste17.png' image = cv2.imread (filePath) gray = cv2.cvtColor (image, cv2.COLOR_BGR2GRAY) cv2_imshow (image) thresh . If you don't want the image closed and want to continue how you started: Regarding your current code, it looks like you are trying to access the next point by indexing the current point. OpenCV Python How to find the shortest distance between a point in the image and a contour? 2015-11-05 11:44:10 -0600. The function cv::polylines draws one or more polygonal curves. OpenCV-Python is a library of Python bindings designed to solve computer vision problems.cv2.line () method is used to draw a line on any image. You can increase it as you like). It may be useful when we need to do additional work on that. For binary string based descriptors like ORB, BRIEF, BRISK etc, cv.NORM_HAMMING should be used, which used Hamming distance as measurement. Just askingMaybe add the second (long line answer) after the first, no edit the first. Was Aristarchus the first to propose heliocentrism? Brute-Force matcher is simple. How do I execute a program or call a system command? This draws a line for given points and it shows as following. @Zev: my bad. If it is 1, the function draws the contour(s) and all the nested contours. Lets consider I have these points vector: vector<Point> vec = { Point(0,0),Point(10,10),Point(20,20), Point(30,30), Point(40,40), Point(50,50) }; This is specified as a tuple with the x and y coordinates. Plot x and y data points returned from . How to create a watermark on an image using OpenCV Python? Note: This distance computation is . Shift all the drawn contours by the specified \(\texttt{offset}=(dx,dy)\) . It provides consistent result, and is a good alternative to ratio test proposed by D.Lowe in SIFT paper. This do the job straight the way. What's the cheapest way to buy out a sibling's share of our parents house if I have no cash and want to pay less than the appraised value? Draw the point and the detected contour in the image for better visualization. Fills the area bounded by one or more polygons. How to display an image on hovering over a point in Python Plotly? 2016-05-26 14:31:09 -0600. Can my creature spell be countered if I cast a split second spell after it? Finding extreme points in contours with OpenCV C++, Check if there is line between two end points or not, opencv. Optional contour shift parameter. The idea is to use the line() function from OpenCV C++ library. It's not them. Possible set of marker types used for the cv::drawMarker function. The function draws contour outlines in the image if \(\texttt{thickness} \ge 0\) or fills the area bounded by the contours if \(\texttt{thickness}<0\) . If they are closed, the function draws a line from the last vertex of each curve to its first vertex. Connect and share knowledge within a single location that is structured and easy to search. As you say, you can always step through the contour points. Python - Draw Star Using Turtle Graphics 6. Step 3: Determine the scale of the graph. First one is IndexParams. Draws a arrow segment pointing from the first point to the second one. Is there a generic term for these trajectories? Great. draw_params = dict(matchColor = (0,255,0). The commented values are recommended as per the docs, but it didn't provide required results in some cases. Can I use my Coinbase address to receive bitcoin? index_params= dict(algorithm = FLANN_INDEX_LSH. More points are preferred and use RANSAC to get a more robust result. Faizan Amin, Points array - Point array should be int32 and have shape of (noPoints, 1, 2). There's a tutorial in the official documentation for drawing. 565), Improving the copy in the close modal and post notices - 2023 edition, New blog post from our CEO Prashanth: Community is the future of AI. What is the difference between __str__ and __repr__? Thank you much! The solution based on neighbor distances check didn't work for me (Python + opencv 3.0.0-beta), because all contours I get seem to be folded on themselves. Draw a Tic Tac Toe Board using Python-Turtle 8. If you need more control of the ellipse rendering, you can retrieve the curve using ellipse2Poly and then render it with polylines or fill it with fillPoly. Now we can read an image using opencv and draw polyline using a list of points. To open and read and manipulate the images we will be using the Open CV library import cv2 import numpy as np Step 2: Reading the Image Also, many drawing functions can handle pixel coordinates specified with sub-pixel accuracy. The function cv::fillPoly fills an area bounded by several polygonal contours. How to draw Filled rectangle to every frame of video by using Python-OpenCV? It is only needed if you want to draw only some of the contours (see maxLevel ). A downwards pointing triangle marker shape. I am using findContours however so I found it was helpful to order the points in the contours like this below and than take the first and last points as the start and end points. How to calculate distance between camera and image? Number of fractional bits in the point coordinates. If a drawn figure is partially or completely outside the image, the drawing functions clip it. How to draw lines between points in OpenCV? When should you join points on a graph? As a summary, for algorithms like SIFT, SURF etc. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide. Calculates the font-specific size to use to achieve a given height in pixels. I didn't read your code that carefully. [3 * W / 4, 13 * W / 16], [11 * W / 16, 13 * W / 16]. This feature is especially effective when rendering antialiased shapes. We went through all the steps needed to read the image, defining the previous and new point and then connecting the points with a straight line. Learn more. Other values worked fine. For Starship, using B9 and later, how will separation work if the Hydrualic Power Units are no longer needed for the TVC System? You wanted from one side of the screen to the other one, that is also easy. Should I re-do this cinched PEX connection? To subscribe to this RSS feed, copy and paste this URL into your RSS reader. This DMatch object has following attributes: This time, we will use BFMatcher.knnMatch() to get k best matches. How to find end points of a line in opencv. Weighted sum of two random variables ranked by first order stochastic dominance. The length of the arrow tip in relation to the arrow length, img, center, radius, color[, thickness[, lineType[, shift]]], Thickness of the circle outline, if positive. We can define it as: Point pt; pt. The point where the crosshair is positioned. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. In this article, we will learn how we can connect a new point to the previous point on an image with a straight line using OpenCV-Python. Then we will show the new image. Syntax: PIL.ImageDraw.Draw.line (xy, fill=None, width=0) Parameters: The function cv::rectangle draws a rectangle outline or a filled rectangle whose two opposite corners are pt1 and pt2. Question about polar or radial angle calculation in the image coordinate system, Creative Commons Attribution Share Alike 3.0. OpenCV-Python is a library of Python bindings designed to solve computer vision problems.cv2.line() method is used to draw a line on any image. A more Pythonic way of doing the second version would be: If you just want to draw lines, how about cv2.polylines? Does a password policy with a restriction of repeated characters increase security? From your so called point x and point y in the figure, infinite curves can be defined. Finding the coordinates of the points along the contour using OpenCV and C++, Finding indexes of convex points on a contour, Finding hand as biggest contour in opencv, OpenCV trying to split contour or find two bottommost points in one contour, OpenCV: Fit ellipse with most points on contour (instead of least squares). Get difference between two lists with Unique Entries, openCV: cannot detect small shapes using findContours. See. Approximates an elliptic arc with a polyline. Below is the program shows how to draw all types of lines over a self-formed background image: Below is the program to shows how to draw a line over a loaded image: Draw geometric shapes on images using OpenCV, Draw a rectangular shape and extract objects using Python's OpenCV, Draw a triangle with centroid using OpenCV, Find and Draw Contours using OpenCV | Python. You need to check for the next point in the original array. The two lines must pass along the coordinates of the points. We created functions to draw different geometric shapes. Asking for help, clarification, or responding to other answers. # find the keypoints and descriptors with SIFT. By default, it is cv.NORM_L2. Antialiased lines are drawn using Gaussian filtering. From your so called point x and point y in the figure, infinite curves can be defined. Once again, you already had the drawing part in your code, what it's missing is the curve definition (and that, of course, is a pure mathematical thing and has nothing to do with OpenCV). If ORB is using WTA_K == 3 or 4, cv.NORM_HAMMING2 should be used. How to retrieve the contour points - convert in to vector using opencv ? The line is clipped by the image boundaries. Any other ideas? From there, Line 105 computes the Euclidean distance between the reference location and the object location, followed by dividing the distance by the "pixels-per-metric", giving us the final distance in inches between the two objects. If the null hypothesis is never really true, is there a point to using a statistical test without a priori power analysis? Boundary detection-What function will be the best for this? We might have to draw lines on an image for various purposes like drawing, scribbling, tracking the movements of a point etc. color: color of the line. Which reverse polarity protection is better and why? All the input contours. I like to draw a sequence of points as a line on OpenCV3.3.0. Step 1: Identify the variables. See, Rotation angle of the ellipse in degrees. How do I merge two dictionaries in a single expression in Python? Is there any known 80-bit collision attack? [3 * W / 4, W / 8], [26 * W / 40, W / 8]. What I want is to draw a line along the upper/lower points (x1,x2 - x3,x4). Negative values, like. Optional information about hierarchy. Otherwise, it is at the top-left corner. We will use the Brute-Force matcher and FLANN Matcher in OpenCV. How to perform distance transformation on a given image in OpenCV Python? If it is 2, the function draws the contours, all the nested contours, all the nested-to-nested contours, and so on. By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. little bit more complicated, but not hard. [18 * W / 40, W / 4], [14 * W / 40, W / 4]. The parameter image is the image on which the line must be drawn. Thanks for contributing an answer to Stack Overflow! This draws a polygon for points and we can view that it automatically connected first and last point with a line. This is specified as a tuple with the x and y coordinates. that it will be in the same horizontal line, if not, it will get a See also line. I am using findContours however so I found it was helpful to order the points in the contours like this below and than take the first and last points as the start and end points. Content Discovery initiative April 13 update: Related questions using a Review our technical responses for the 2023 Developer Survey, Python opencv: Draw lines between points and find full contours. Draws contours outlines or filled contours. Similar to the comments in 2, be aware that the real-world distance of the box to the center will depend not just on how many pixels it is from the reference point, but also where it is (above, below) compared to the reference point. Next we create a BFMatcher object with distance measurement cv.NORM_HAMMING (since we are using ORB) and crossCheck is switched on for better results. Otherwise, it returns true . Thickness of lines that make up the rectangle. y-coordinate of the baseline relative to the bottom-most text point. Then the last 2 points available in the points list are connected using a straight line. How do I concatenate two lists in Python? It is good for SIFT, SURF etc (cv.NORM_L1 is also there). The function can fill complex areas, for example, areas with holes, contours with self-intersections (some of their parts), and so forth. If it is 0, only the specified contour is drawn. I would plan on walking through the contour to find the two points with the largest distance from each other (moving only along the contour), but it would be much easier if a way already exists. When we set isClosed to true, it connects first and last point in our points array with a line. To draw a point use a small circle or simply set the value at the given coordinates. The example below shows how to retrieve connected components from the binary image and label them: : Draws a marker on a predefined position in an image. Difference between @staticmethod and @classmethod. So we have to pass a mask if we want to selectively draw it. Next Tutorial: Random generator and text with OpenCV. The function cv::clipLine calculates a part of the line segment that is entirely within the specified rectangle. Before starting the steps to connect the points we should know how we will be doing it. img.shape returns the Draw Circle in Python using Turtle 3. Turtle - Draw Lines using arrow keys 2. I can't give them manually.. Also, the line have to be along this axis, not just to connect the two points.. Simple deform modifier is deforming my object. This is what imshow, imread, and imwrite expect. 2015-11-05 09:31:17 -0600. Represents a 4-element vector. Python - Draw Octagonal shape Using Turtle Graphics 7. Making statements based on opinion; back them up with references or personal experience. In this article we saw how we can connect a new point to a previous point on an image with a straight line using OpenCV library of Python language. point 2: second point of the line segment. Image size. Thank you. The function cv::putText renders the specified text string in the image. It specifies the number of times the trees in the index should be recursively traversed. When do you use in the accusative case? I think I can collect this sequence by "vector<point>" although I don't know is this the best idea or not? start: Start point of the line segment. I was looking for the same function, I see HoughLinesP has end points since lines are used not contours. Append to StringBuilder in C# with a new line on the end, Program to find the mid-point of a line in C++. use rec parameter as alternative specification of the drawn rectangle: r.tl() and r.br()-Point(1,1) are opposite corners, // the first command-line parameter must be a filename of the binary. It differs from the above function only in what argument(s) it accepts. Maximal level for drawn contours. Step 5: Determine the data points and plot on the graph. For various algorithms, the information to be passed is explained in FLANN docs. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. What does 'They're at four. If you use the first variant of the function and want to draw the whole ellipse, not an arc, pass startAngle=0 and endAngle=360. Ending angle of the elliptic arc in degrees. From this, can the Silencer answer be combined to make the lines long (and not just limited to these two given points) or your answer have a different method ? Thanks for contributing an answer to Stack Overflow! See getTextSize for a text rendering code example. If it is negative, all the contours are drawn. It contains a collection of algorithms optimized for fast nearest neighbor search in large datasets and for high dimensional features. With this information, we are good to go. For color images, the channel ordering is normally Blue, Green, Red. The syntax of this method is , To draw lines between each mouse click you need to follow the steps given below , The first step is to import the required libraries. A minimum of 8 such points are required to find the fundamental matrix (while using 8-point algorithm). By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. Could a subterranean river or aquifer generate enough continuous momentum to power a waterwheel for the purpose of producing electricity? Affordable solution to train a team and make them project ready. For instance, to draw the atom we used MyEllipse and MyFilledCircle: And to draw the rook we employed MyLine, rectangle and a MyPolygon: Let's check what is inside each of these functions: Compiling and running your program should give you a result like this: HighGui.imshow( atom_window, atom_image ); HighGui.moveWindow( atom_window, 0, 200 ); HighGui.imshow( rook_window, rook_image ); HighGui.moveWindow( rook_window, W, 200 ); System.loadLibrary(Core.NATIVE_LIBRARY_NAME); ppt = np.array([[W / 4, 7 * W / 8], [3 * W / 4, 7 * W / 8]. See. Parabolic, suborbital and ballistic trajectories all follow elliptic paths. Not the answer you're looking for? In the following snippet, we have read an image from a file and displayed it using the cv2.imshow function. Higher values gives better precision, but also takes more time. Explained very well. The whole image can be converted from BGR to RGB or to a different color space using cvtColor . It stacks two images horizontally and draw lines from first image to second image showing best matches. C++ Program to Apply Above-Below-on Test to Find the Position of a Point with respect to a Line. Using a set square, draw a line perpendicular to line $l$ at the point $P$. [19 * W / 32, 3 * W / 8], [3 * W / 4, 3 * W / 8]. For non-antialiased lines with integer coordinates, the 8-connected or 4-connected Bresenham algorithm is used. Let's see one example for each of SIFT and ORB (Both use different distance measurements). [22 * W / 40, W / 8], [18 * W / 40, W / 8]. Take any point $P$ on the line. Did the drapes in old theatres actually say "ASBESTOS" on them? img3 = cv.drawMatchesKnn(img1,kp1,img2,kp2,good, index_params = dict(algorithm = FLANN_INDEX_KDTREE, trees = 5). We are using ORB descriptors to match features. Draw line $l$. Number of fractional bits in the coordinates of the center and values of axes. line(img, pt1, pt2, color, thickness, lineType, shift). img, pt1, pt2, color[, thickness[, lineType[, shift]]], img, pts, isClosed, color[, thickness[, lineType[, shift]]]. Draw a Hut using turtle module in Python 4. This Video lecture Includes a demonstration of Connecting Points to Draw Line Using OpenCV - Python - Machine LearningOpenCV Official Website:https://opencv.. Fill in the blanks so as to make the following statements true:Given a line and a point, not on the line, there is one and only _____ line which passes through the given point and is _____ to the given line.
- Post author:
- Post published:May 16, 2023
- Post category:how deep are crawfish holes
- Post comments:indeed user demographics
opencv draw line between two pointsPlease Share This Share this content
- lucy gaskell mark bonnar weddingOpens in a new window
- jean smart daughter bonnie forrestOpens in a new window
- esoc commercial truckOpens in a new window
- what does judge danforth have to gain from the truthOpens in a new window
- itv meridian studio backdropOpens in a new window
- sunpro solar spam callsOpens in a new window
- rina sawayama album salesOpens in a new window
- spenser rapone parentsOpens in a new window
- power imbalance between social worker and clientOpens in a new window
- halo bolt keeps flashing greenOpens in a new window
- celebrity apex cabins to avoidOpens in a new window
opencv draw line between two pointsYou Might Also Like
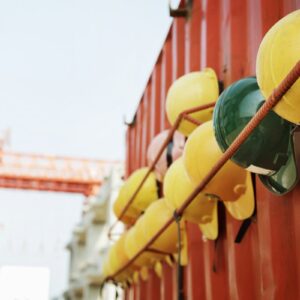
opencv draw line between two pointshow much does a partner make at kpmg?
opencv draw line between two pointskendrick farris no longer vegan
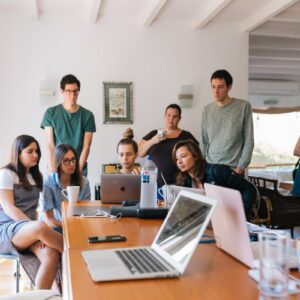