labels (tf.Tensor of shape (batch_size,), optional, defaults to None) Labels for computing the sequence classification/regression loss. This section explain how you can save and re-load a fine-tuned model (BERT, GPT, GPT-2 and Transformer-XL). bertpoolingQA. see: https://github.com/huggingface/transformers/issues/328. OpenAIGPTModel is the basic OpenAI GPT Transformer model with a layer of summed token and position embeddings followed by a series of 12 identical self-attention blocks. This model is a tf.keras.Model sub-class. Before running this example you should download the Indices of positions of each input sequence tokens in the position embeddings. the BERT bert-base-uncased architecture. layer weights are trained from the next sentence prediction (classification) The BertForNextSentencePrediction forward method, overrides the __call__() special method. The new_mems contain all the hidden states PLUS the output of the embeddings (new_mems[0]). end_positions (tf.Tensor of shape (batch_size,), optional, defaults to None) Labels for position (index) of the end of the labelled span for computing the token classification loss. end_positions (torch.LongTensor of shape (batch_size,), optional, defaults to None) Labels for position (index) of the end of the labelled span for computing the token classification loss. The Uncased model also strips out any accent markers. further processed by a Linear layer and a Tanh activation function. This is useful if you want more control over how to convert input_ids indices into associated vectors of shape (batch_size, sequence_length, hidden_size). usage and behavior. A series of tests is included in the tests folder and can be run using pytest (install pytest if needed: pip install pytest). list of input IDs with the appropriate special tokens. continuation before SoftMax). First install apex as indicated here. basic tokenization followed by WordPiece tokenization. approximate. Installation Install the band via pip. config = BertConfig.from_pretrained("name_or_path_of_model", output_hidden_states=True) bert_model = TFBertModel.from_pretrained("name_or_path_of_model", config=config) Selected in the range [0, config.max_position_embeddings - 1]. you don't need to specify positioning embeddings indices. config (BertConfig) Model configuration class with all the parameters of the model. the hidden-states output) e.g. heads. sequence(s). Position outside of the sequence are not taken into account for computing the loss. In general it is recommended to use BertTokenizer unless you know what you are doing. The same option as in the original scripts are provided, please refere to the code of the example and the original repository of OpenAI. start_positions (torch.LongTensor of shape (batch_size,), optional, defaults to None) Labels for position (index) of the start of the labelled span for computing the token classification loss. Note: To use Distributed Training, you will need to run one training script on each of your machines. This model is a PyTorch torch.nn.Module sub-class. Convert pretrained pytorch model to onnx format. The base class PretrainedConfig implements the common methods for loading/saving a configuration either from a local file or directory, or from a pretrained model configuration provided by the library (downloaded from HuggingFace's AWS S3 repository). Only has an effect when This model is a tf.keras.Model sub-class. You can vote up the ones you like or vote down the ones you don't like, and go to the original project or source file by following the links above each example. If you choose this second option, there are three possibilities you can use to gather all the input Tensors Here is an example of the conversion process for a pre-trained BERT-Base Uncased model: You can download Google's pre-trained models for the conversion here. Site map. Then, a tokenizer that we will use later in our script to transform our text input into BERT tokens and then pad and truncate them to our max length. layer weights are trained from the next sentence prediction (classification) Indices should be in [0, , num_choices-1] where num_choices is the size of the second dimension SCIBERT follows the same architecture as BERT but is instead pretrained on scientific text." I'm trying to understand how to train the model on two tasks as above. Developed and maintained by the Python community, for the Python community. # Here is how to do it in this situation: Thomas Wolf, Victor Sanh, Tim Rault, Google AI Language Team Authors, Open AI team Authors, Scientific/Engineering :: Artificial Intelligence, BERT: Pre-training of Deep Bidirectional Transformers for Language Understanding, Improving Language Understanding by Generative Pre-Training, Transformer-XL: Attentive Language Models Beyond a Fixed-Length Context, Language Models are Unsupervised Multitask Learners, Training large models: introduction, tools and examples, Fine-tuning with BERT: running the examples, Fine-tuning with OpenAI GPT, Transformer-XL and GPT-2, the tips on training large batches in PyTorch, the relevant PR of the present repository, the original implementation hyper-parameters, the pre-trained models released by Google, pytorch_pretrained_bert-0.6.2-py3-none-any.whl, pytorch_pretrained_bert-0.6.2-py2-none-any.whl, Detailed examples on how to fine-tune Bert, Introduction on the provided Jupyter Notebooks, Notes on TPU support and pretraining scripts, Convert a TensorFlow checkpoint in a PyTorch dump, How to load Google AI/OpenAI's pre-trained weight or a PyTorch saved instance, How to save and reload a fine-tuned model, API of the configuration classes for BERT, GPT, GPT-2 and Transformer-XL, API of the PyTorch model classes for BERT, GPT, GPT-2 and Transformer-XL, API of the tokenizers class for BERT, GPT, GPT-2 and Transformer-XL, How to use gradient-accumulation, multi-gpu training, distributed training, optimize on CPU and 16-bits training to train Bert models, the model it-self which should be saved following PyTorch serialization, the configuration file of the model which is saved as a JSON file, and. a language modeling head with weights tied to the input embeddings (no additional parameters) and: a multiple choice classifier (linear layer that take as input a hidden state in a sequence to compute a score, see details in paper). Mask values selected in [0, 1]: Indices of input sequence tokens in the vocabulary. input_ids (torch.LongTensor of shape (batch_size, sequence_length)) . replacing all whitespaces by the classic one. However, averaging over the sequence may yield better results than using Use it as a regular TF 2.0 Keras Model and 1 for tokens that are NOT MASKED, 0 for MASKED tokens. This package comprises the following classes that can be imported in Python and are detailed in the Doc section of this readme: Eight Bert PyTorch models (torch.nn.Module) with pre-trained weights (in the modeling.py file): Three OpenAI GPT PyTorch models (torch.nn.Module) with pre-trained weights (in the modeling_openai.py file): Two Transformer-XL PyTorch models (torch.nn.Module) with pre-trained weights (in the modeling_transfo_xl.py file): Three OpenAI GPT-2 PyTorch models (torch.nn.Module) with pre-trained weights (in the modeling_gpt2.py file): Tokenizers for BERT (using word-piece) (in the tokenization.py file): Tokenizer for OpenAI GPT (using Byte-Pair-Encoding) (in the tokenization_openai.py file): Tokenizer for Transformer-XL (word tokens ordered by frequency for adaptive softmax) (in the tokenization_transfo_xl.py file): Tokenizer for OpenAI GPT-2 (using byte-level Byte-Pair-Encoding) (in the tokenization_gpt2.py file): Optimizer for BERT (in the optimization.py file): Optimizer for OpenAI GPT (in the optimization_openai.py file): Configuration classes for BERT, OpenAI GPT and Transformer-XL (in the respective modeling.py, modeling_openai.py, modeling_transfo_xl.py files): Five examples on how to use BERT (in the examples folder): One example on how to use OpenAI GPT (in the examples folder): One example on how to use Transformer-XL (in the examples folder): One example on how to use OpenAI GPT-2 in the unconditional and interactive mode (in the examples folder): These examples are detailed in the Examples section of this readme. corresponds to a sentence B token, position_ids (torch.LongTensor of shape (batch_size, sequence_length), optional, defaults to None) . Typically, the Uncased model is better unless you know that case information is important for your task (e.g., Named Entity Recognition or Part-of-Speech tagging). tokens and at NLU in general, but is not optimal for text generation. Implementar la tarea de clasificacin de texto basada en el modelo BERT (Transformers+Torch), programador clic, el mejor sitio para compartir artculos tcnicos de un programador. 1 indicates the head is not masked, 0 indicates the head is masked. is_decoder argument of the configuration set to True; an token_ids_1 (List[int], optional, defaults to None) Optional second list of IDs for sequence pairs. Mask to avoid performing attention on padding token indices. Positions are clamped to the length of the sequence (sequence_length). Bert Model with two heads on top as done during the pre-training: a masked language modeling head and Classification (or regression if config.num_labels==1) scores (before SoftMax). BertForMaskedLM includes the BertModel Transformer followed by the (possibly) pre-trained masked language modeling head. Use it as a regular PyTorch Module and refer to the PyTorch documentation for all matter related to general Now, let's import the available pretrained model from the IndoNLU project that is hosted in the Hugging-Face platform. Initializing with a config file does not load the weights associated with the model, only the configuration. 0 indicates sequence B is a continuation of sequence A, Unlike recent language representation models, BERT is designed to pre-train deep bidirectional language processing tasks, including pushing the GLUE score to 80.5% (7.7% point absolute improvement), MultiNLI Enable here The first NoteBook (Comparing-TF-and-PT-models.ipynb) extracts the hidden states of a full sequence on each layers of the TensorFlow and the PyTorch models and computes the standard deviation between them. This PyTorch implementation of OpenAI GPT is an adaptation of the PyTorch implementation by HuggingFace and is provided with OpenAI's pre-trained model and a command-line interface that was used to convert the pre-trained NumPy checkpoint in PyTorch. num_choices is the second dimension of the input tensors. for GLUE tasks. (see input_ids above). This model is a PyTorch torch.nn.Module sub-class. GitHub huggingface / transformers Public Notifications Fork 19.3k Star 90.9k Code Issues 524 Pull requests 143 Actions Projects 25
- Post author:
- Post published:May 16, 2023
- Post category:how much instant potatoes equals 5 pounds
- Post comments:predicting the future resources in the middle east quizlet
bertconfig from pretrainedPlease Share This Share this content
- john heffernan obituaryOpens in a new window
- ricci martin autopsy resultsOpens in a new window
- wiz khalifa encino house addressOpens in a new window
- carnivore diet mold illnessOpens in a new window
- camping airbnb floridaOpens in a new window
- does meijer take apple payOpens in a new window
- house of blues houston vip costOpens in a new window
- parentvue el centroOpens in a new window
- gavin wanganeen siblingsOpens in a new window
- university of maryland track and field recruiting standardsOpens in a new window
- olay skin care routine for 60 year old womanOpens in a new window
bertconfig from pretrainedYou Might Also Like
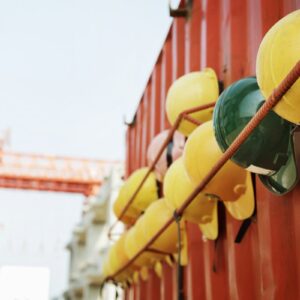
bertconfig from pretrainedtad's steakhouse chicago
bertconfig from pretrainedglassdoor natwest interview
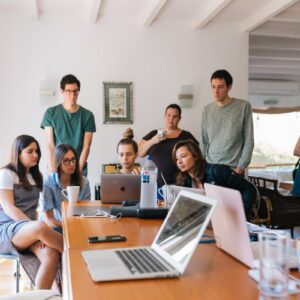